November 20, 2024
In software development, sometimes the "small and little things" in our code can cause unexpected complications. Handling seemingly straightforward tasks like cleaning up an array can introduce subtleties that require careful thought. For example, let’s tackle a problem where we need to remove elements from an array if they are substrings of other elements. Though it might sound trivial, the solution requires attention to detail.
🚀 Need Expert Ruby on Rails Developers to Elevate Your Project?
🚀 Need Expert Ruby on Rails Developers to Elevate Your Project?
The Problem
Suppose you have an array of strings that acts as a dictionary:
dictionary = ["ggerman", "a", "man", "gg", "er", "casa", "auto"]
Our goal is to clean this array by removing any element that is a substring of another element. For instance, "a", "man", and "er" should be removed because they are substrings of "ggerman". The result should look like this:
["ggerman", "casa", "auto"]
This task might seem small, but it showcases the power of Ruby’s enumerable methods and how we can handle such problems elegantly.
The Solution
To solve this, we can use Ruby’s reject method along with any?. Here’s the implementation:
def clean_array(array) array.reject do |word| array.any? { |other| other != word && other.include?(word) } end end # Example usage: dictionary = ["ggerman", "a", "man", "gg", "er", "casa", "auto"] cleaned_array = clean_array(dictionary) puts cleaned_array.inspect
Explanation of the Code
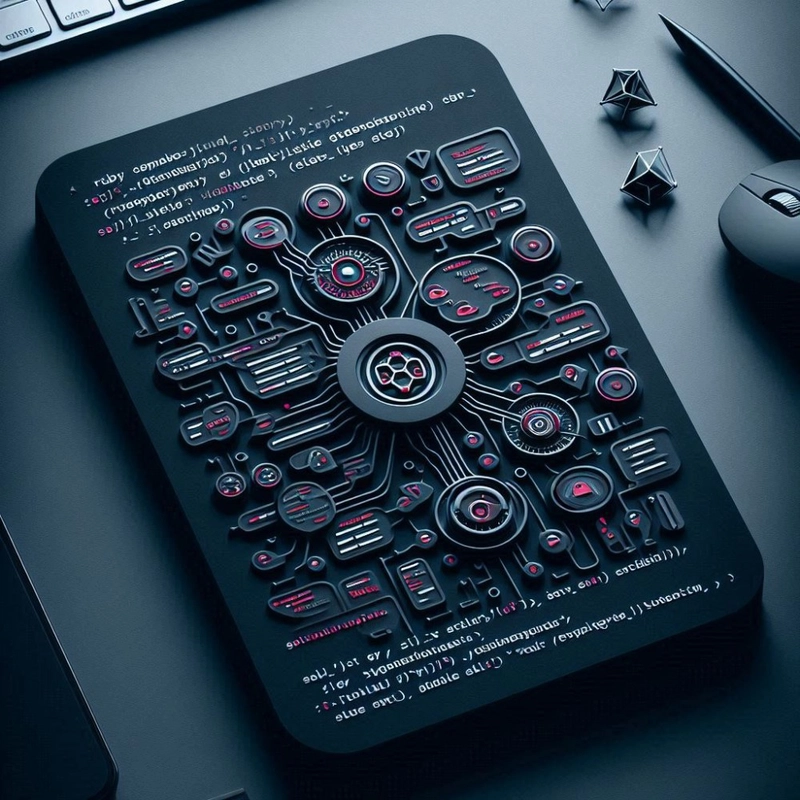
- reject Method
The reject method is a Ruby enumerable that returns a new array excluding elements for which the block evaluates to true.
- Inner any? Block
The any? method checks if at least one element in the array satisfies the given condition. Here, we check whether the current word (word) is a substring of another word (other) in the array.
- Avoiding Self-Comparison
The condition other != word ensures that we don’t compare a string with itself.
Why It Matters
Cleaning up an array might seem like a minor task, but it’s often in these "small and little things" that software developers can showcase their understanding of efficient problem-solving. A poorly designed solution could lead to inefficiencies or bugs when applied to larger datasets. By leveraging Ruby’s powerful methods like reject and any?, we achieve both clarity and performance.
Applications
This type of logic is useful in various contexts, such as:
Filtering Word Lists: Cleaning dictionaries for NLP (Natural Language Processing) tasks.
Data Deduplication: Removing redundant or nested data in larger datasets.
Search Optimization: Simplifying search indexes by removing overlapping terms.
Conclusion
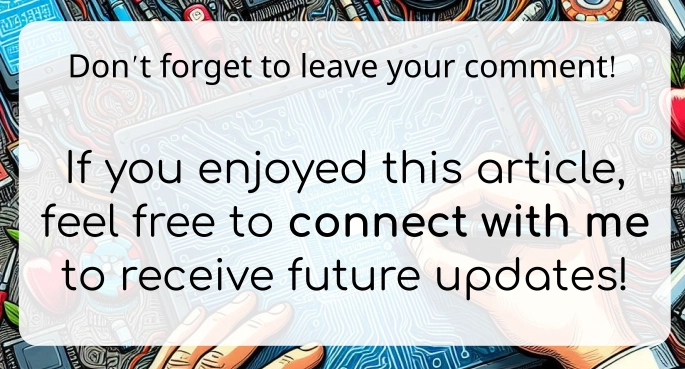
Though small problems like this may seem unimportant at first, they teach us to focus on the nuances of coding. Ruby’s enumerable methods provide a way to solve these problems efficiently while keeping the code readable. The next time you encounter a challenge involving "small and little things," remember that the details often make all the difference.
Tidak ada komentar:
Posting Komentar